thjvjpxz learn-design-pattern-java
Table Of Content
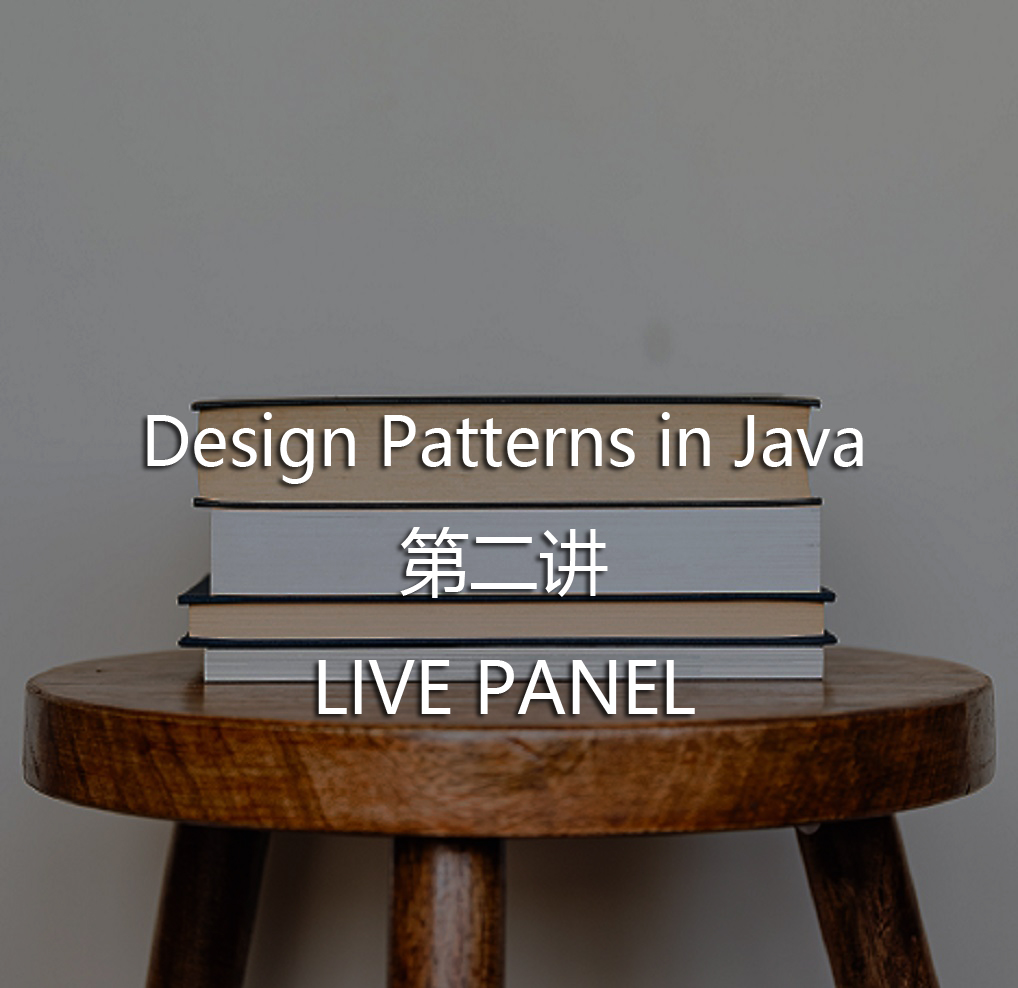
The State pattern is a behavioral design pattern that lets an object alter its behavior when its internal state changes. We define a SortingStrategy interface (strategy) with a sort() method. The BubbleSortStrategy and QuickSortStrategy classes (concrete strategies) implement the SortingStrategy interface and provide their respective sorting algorithms.
Strategy Design Pattern and Open Closed Principle in Java - Example
Next, we ventured into expert-level territory, introducing alternative approaches such as the Composite and State patterns. We provided code examples for these patterns, discussed their advantages and disadvantages, and provided recommendations for their use. This pattern ensures that a class only has one instance and provides a global point of access to it. In conclusion, while design patterns in Java are powerful tools, they must be used judiciously and appropriately. Understanding the potential issues and how to avoid them will help you use design patterns more effectively in your Java projects. We define three subsystem classes, SubsystemA, SubsystemB, and SubsystemC, each with their respective operation methods.
components
In this article, we had a quick look over a variety of design patterns. If we look at it as object-oriented code, the driver class is in control of the customer class. The driver class can add new operations on top of the customer/visitor.
Visitor Design Pattern in Java
The 7 Software Architecture Books Experienced Developers Need to Read - hackernoon.com
The 7 Software Architecture Books Experienced Developers Need to Read.
Posted: Thu, 03 Aug 2023 07:00:00 GMT [source]
In case you need to define an additional speed level, just add in a new class that implements the SpeedLevel interface and implement its rotate method. You have a set of elements arranged in a collection, and you want to sequentially access those elements. A good example of an Iterator is a TV remote, which has the “next” and “previous” buttons to surf TV channels. Pressing the “next” button takes me one channel in the forward direction, and pressing the “previous” button takes me one channel in the backward direction. The drawback of this approach is the complexity involved in creating objects.

What is the Visitor Design Pattern?
Lets you ensure that a class has only one instance, while providing a global access point to this instance. Null Object Method is a Behavioral Design Pattern, it is used to handle the absence of a valid object by providing an object that does nothing or provides default behavior. State Method is a Behavioral Design Pattern, it allows an object to alter its behavior when its internal state changes.
Visitor Interface
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It's useful when you have multiple ways to solve a problem and want to choose an algorithm at runtime. Sometimes, developers force the use of design patterns where they’re not needed, leading to unnecessary complexity.
Design patterns are the best formalized practices a programmer can use to solve common problems when designing an application or system. The Chain of Responsibility pattern allows you to pass requests along a chain of handlers. Each handler decides whether to process the request or pass it to the next handler in the chain. The Flyweight pattern uses sharing to support large numbers of fine-grained objects efficiently. It's useful when you have a large number of objects that share a common state and can be replaced by a single shared object.
Use Cases of the Visitor Design Pattern
The idea is to keep the service layer separate from the data access layer. The singleton pattern restricts the instantiation of a Class and ensures that only one instance of the class exists in the Java Virtual Machine. The implementation of the singleton pattern has always been a controversial topic among developers. A design pattern is a well-described solution to a common software problem.
Factory Method
The Prototype pattern specifies the kind of object to create using a prototypical instance and creates new objects by cloning this prototype. Use the FactoryProducer to get AbstractFactory in order to get factories of concrete classes by passing an information such as type. We are demonstrating the use of decorator pattern via following example in which we will decorate a shape with some color without alter shape class.
In object-oriented programming, we typically use a lot of inheritance. If somebody wants to create a male person, they invoke the getPerson() method on the PersonFactory with a gender argument of "M". Similarly, you can create a female person by invoking the getPerson() method on the PersonFactory with a gender argument of "F". Whenever a new chess game is started, for example, in any of the numerous online Chess portals, this initialized instance is merely copied or cloned.
The VectorRenderer and RasterRenderer classes (concrete implementors) implement the Renderer interface and override the renderCircle() method. The dependency injection pattern allows us to remove the hard-coded dependencies and make our application loosely-coupled, extendable, and maintainable. We can implement dependency injection in Java to move the dependency resolution from compile-time to runtime. Spring framework is built on the principle of dependency injection. The command pattern is used to implement loose-coupling in a request-response model.
This is useful when you need a single object to coordinate actions across the system. For instance, overusing the Factory pattern can lead to an explosion of factory classes, making the code harder to understand and manage. The Singleton pattern is a design pattern that restricts the instantiation of a class to a single instance. This is useful when exactly one object is needed to coordinate actions across the system. We define an abstract Graphic class with a draw() method and add() and remove() methods that throw UnsupportedOperationException by default. The Circle class (leaf) extends Graphic and overrides the draw() method.
The Circle class (concrete flyweight) implements the Shape interface and has a color property. The ShapeFactory class provides a static getCircle() method that returns a Circle instance with the specified color. If a circle with the given color doesn't exist, it creates a new one, stores it in the shapeMap, and returns it.
Comments
Post a Comment